Speed Up Your Python Web Scraping: Techniques & Tools
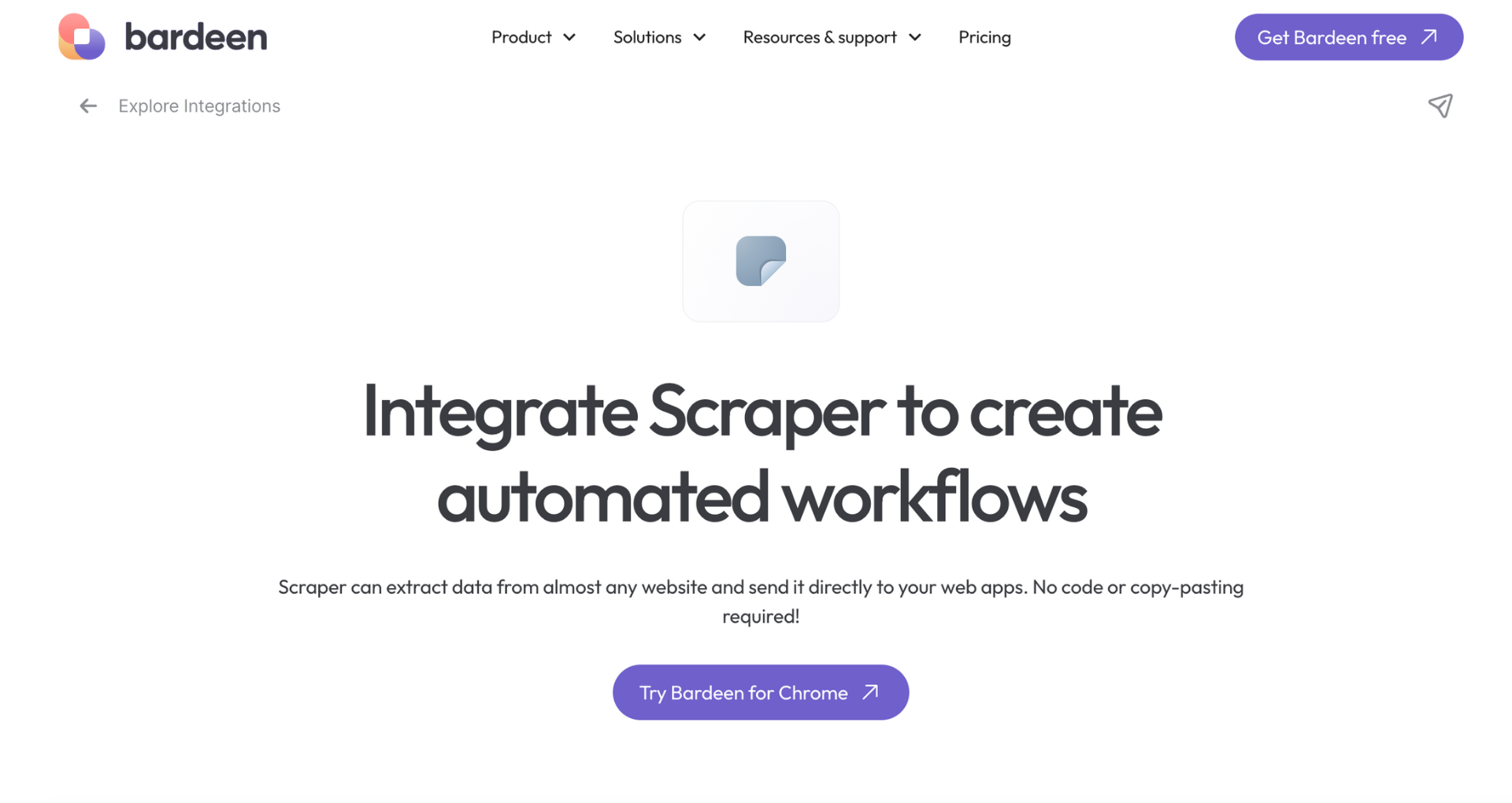
TL;DR
To web scrape faster, employ multiprocessing, multithreading, asyncio, and tools like Browse AI for no-code solutions. Efficient scraping also involves minimizing unnecessary requests and using strategies for CAPTCHAs and dynamic content.
These methods enhance speed and efficiency in data collection.
Automate your web scraping tasks efficiently with Bardeen and save time on manual data collection.
Web scraping is an essential tool for data-driven industries, enabling businesses to gather valuable insights from online sources. However, the process of extracting data from websites can be time-consuming and challenging, especially when dealing with large volumes of data and dynamic content. In this blog post, we will explore essential techniques and best practices to boost your web scraping speed, helping you optimize your data collection efforts and achieve faster results.
Introduction
Web scraping has become an indispensable tool for businesses and individuals looking to extract valuable data from websites. Whether you're gathering market intelligence, monitoring competitor prices, or collecting data for research purposes, web scraping enables you to automate the process and save time. However, as websites grow more complex and the volume of data increases, the speed of your web scraping operations becomes crucial.
Slow web scraping can lead to several issues, such as increased server load, IP blocking, and inefficient use of resources. By optimizing your web scraping techniques and adopting best practices, you can significantly boost your scraping speed and achieve faster data collection. In this blog post, we will explore essential techniques and strategies to help you streamline your web scraping process and maximize efficiency.
We'll cover topics such as:
- Understanding the basics of web scraping and common challenges
- Optimizing web scraping with multithreading and multiprocessing
- Leveraging asynchronous programming with Python's Asyncio
- Advanced techniques like proxy rotation and session management
- Tools and frameworks to streamline your web scraping projects
By implementing these techniques and best practices, you'll be able to extract data from websites more efficiently, reduce the risk of being blocked, and ultimately save time and resources in your web scraping endeavors. Let's dive in and explore how you can boost your web scraping speed!
Understanding the Basics of Web Scraping
Web scraping is the process of extracting data from websites automatically using specialized software or scripts. It involves retrieving the HTML content of a web page, parsing it, and extracting the desired information. Web scraping has become increasingly popular in data-driven industries, such as e-commerce, market research, and data analytics, as it enables businesses to gather valuable insights and make data-informed decisions.
The usefulness of web scraping lies in its ability to:
- Collect large amounts of data quickly and efficiently
- Automate repetitive tasks and save time
- Access data that may not be available through APIs or other means
- Monitor competitor prices, product details, and market trends
- Gather data for research and analysis purposes
However, web scraping also comes with its own set of challenges. Some common issues faced during web scraping include:
- Network delays and timeouts: Slow internet connections or server response times can hinder the scraping process and lead to incomplete or inconsistent data collection.
- Handling dynamic content: Many modern websites heavily rely on JavaScript to load content dynamically, making it difficult for traditional scraping techniques to capture the desired data.
- IP blocking and CAPTCHAs: Websites may implement measures to prevent excessive or unauthorized scraping, such as blocking IP addresses or presenting CAPTCHAs to verify human interaction.
- Changes in website structure: Websites may undergo changes in their HTML structure or layout, requiring scraping scripts to be updated and maintained regularly.
To overcome these challenges, web scraping practitioners employ various techniques and best practices, such as using headless browsers, implementing delays between requests, and utilizing proxy servers to avoid IP blocking. Additionally, tools like Selenium and Puppeteer can help handle dynamic content and simulate user interactions.
Use Bardeen's web scraper to collect data effortlessly. Automate routine scraping tasks and save valuable time with our no-code tool.
Understanding the basics of web scraping is crucial for anyone looking to harness the power of data from websites. By being aware of the challenges and adopting the right techniques, you can effectively scrape data while respecting website terms of service and ethical guidelines.
Optimizing Web Scraping with Multithreading
Multithreading is a technique that allows multiple tasks to be executed concurrently within a single program. By leveraging multithreading in web scraping, you can significantly improve the performance and speed of your scraping operations. Instead of sequentially processing each web page, multithreading enables you to handle multiple pages simultaneously, reducing the overall execution time.
Here's how multithreading can be applied to web scraping:
- Create a thread pool: A thread pool is a collection of worker threads that are ready to execute tasks. By creating a thread pool, you can efficiently manage and reuse threads, avoiding the overhead of creating new threads for each task.
- Assign scraping tasks to threads: Divide your scraping tasks into smaller units of work, such as individual web pages or specific sections of a website. Assign each task to a thread from the thread pool, allowing them to be processed concurrently.
- Synchronize data access: When multiple threads are accessing and modifying shared data, it's crucial to implement proper synchronization mechanisms to avoid race conditions and ensure data integrity. Use thread-safe data structures or locks to coordinate access to shared resources.
- Handle network delays: Multithreading can help mitigate the impact of network delays by allowing other threads to continue execution while waiting for a response from a website. This ensures that the scraping process doesn't come to a halt due to slow server responses.
Here's a basic example of how multithreading can be implemented in Python for faster web scraping:
import threading
from queue import Queue
def scrape_page(url):
# Scraping logic goes here
# ...
def worker():
while True:
url = url_queue.get()
scrape_page(url)
url_queue.task_done()
url_queue = Queue()
num_threads = 4
for i in range(num_threads):
t = threading.Thread(target=worker)
t.daemon = True
t.start()
# Add URLs to the queue
for url in urls:
url_queue.put(url)
url_queue.join()
In this example:
- We define a
scrape_page
function that contains the scraping logic for a single web page. - The
worker
function acts as the task executor for each thread. It continuously retrieves URLs from the queue, scrapes the corresponding web page, and marks the task as done. - We create a
Queue
to store the URLs that need to be scraped. - Multiple threads are created based on the
num_threads
variable, and each thread executes theworker
function. - URLs are added to the queue, and the main thread waits for all tasks to be completed using
url_queue.join()
.
By distributing the scraping tasks across multiple threads, you can achieve parallel processing and significantly reduce the overall scraping time. However, it's important to note that the actual performance gain depends on factors such as the number of available cores, network latency, and the website's response time.
Multithreading is particularly useful when the majority of the scraping time is spent waiting for I/O operations, such as network requests. By allowing other threads to continue execution while waiting for a response, you can optimize resource utilization and improve scraping efficiency.
Leveraging Multiprocessing for Enhanced Performance
While multithreading is effective for I/O-bound tasks, multiprocessing is the preferred approach when it comes to CPU-bound operations like web scraping without code. Multiprocessing allows you to utilize multiple CPU cores, enabling true parallel execution of scraping tasks.
Here are the key differences between multithreading and multiprocessing:
- Multithreading runs multiple threads within a single process, sharing the same memory space. It is suitable for I/O-bound tasks where threads spend most of their time waiting for I/O operations to complete.
- Multiprocessing spawns multiple processes, each with its own memory space. It is ideal for CPU-bound tasks that require intensive computation, as each process can run on a separate CPU core.
To set up a multiprocessing environment in Python for faster web scraping, follow these steps:
- Import the necessary modules:
from multiprocessing import Pool import requests from bs4 import BeautifulSoup
- Define a function that performs the scraping task for a single URL:
def scrape_page(url): response = requests.get(url) soup = BeautifulSoup(response.text, 'html.parser') # Extract desired data from the parsed HTML # ...
- Create a pool of worker processes:
pool = Pool(processes=4) # Adjust the number of processes based on your system
- Prepare a list of URLs to be scraped:
urls = [...] # List of URLs to scrape
- Use the
map
function to distribute the scraping tasks among the worker processes:results = pool.map(scrape_page, urls)
- Close the pool and wait for all processes to complete:
pool.close() pool.join()
- Process the scraped data stored in the
results
list.
By leveraging multiprocessing, you can significantly speed up your web scraping tasks, especially when dealing with a large number of URLs. The scraping workload is distributed among multiple processes, allowing for parallel execution and efficient utilization of system resources.
However, it's important to note that using multiprocessing may put a higher load on the target website, so it's crucial to be mindful of the website's terms of service and implement appropriate throttling mechanisms to avoid overloading the server.
Save time with the Bardeen scraper. Automate routine scraping tasks without coding.
Asynchronous Scraping with Asyncio
Asynchronous programming is a powerful approach that can significantly boost the performance of web scraping operations. By leveraging Python's asyncio library and the aiohttp package, you can efficiently scrape multiple web pages concurrently, reducing the overall execution time.
Here's a step-by-step guide on setting up an asynchronous web scraping script using Python's asyncio and aiohttp:
- Install the required libraries:
pip install aiohttp beautifulsoup4
- Import the necessary modules:
import asyncio import aiohttp from bs4 import BeautifulSoup
- Define an asynchronous function to fetch the HTML content of a URL:
async def fetch_html(session, url): async with session.get(url) as response: return await response.text()
- Create an asynchronous function to parse the HTML content using BeautifulSoup:
async def parse_html(html): soup = BeautifulSoup(html, 'html.parser') # Extract desired data from the parsed HTML # ...
- Define the main asynchronous function:
async def main(): async with aiohttp.ClientSession() as session: urls = [...] # List of URLs to scrape tasks = [] for url in urls: task = asyncio.ensure_future(fetch_html(session, url)) tasks.append(task) htmls = await asyncio.gather(*tasks) for html in htmls: await parse_html(html)
- Run the main function using the asyncio event loop:
asyncio.run(main())
By utilizing asyncio and aiohttp, you can send multiple requests concurrently without waiting for each request to complete before moving on to the next. This asynchronous approach allows for efficient utilization of system resources and significantly reduces the overall scraping time.
Keep in mind that while asynchronous scraping can greatly improve performance, it's important to be mindful of the target website's terms of service and implement appropriate rate limiting and throttling mechanisms to avoid overloading the server.
Advanced Techniques: Proxy Rotation and Session Management
When scraping websites at scale, using a single IP address can quickly lead to getting blocked. To avoid this, implementing proxy rotation and proper session management is crucial. Here are some key strategies:
- Use a diverse pool of proxies, including a mix of datacenter and residential IPs, to distribute requests across different IP addresses.
- Rotate proxies regularly, either by assigning a new proxy for each request or by switching proxies after a certain number of requests.
- Implement random delays between requests to mimic human browsing behavior and avoid triggering rate limits.
- Maintain cookies and session information when rotating proxies to ensure a consistent browsing experience and avoid detection.
- Monitor proxy performance and automatically remove or replace proxies that become slow, unresponsive, or get banned.
By effectively managing your proxy pool and sessions, you can significantly reduce the chances of getting blocked while scraping websites. However, it's important to respect website terms of service and adhere to ethical scraping practices.
Some popular tools and libraries that can help with proxy rotation and session management include:
- Python's
requests
library with proxy support - Scrapy's built-in proxy middleware
- Proxy management services like Bright Data or Oxylabs
- Open-source tools like ProxyBroker or Scylla
Implementing proxy rotation and session management requires careful planning and ongoing monitoring to ensure the success and reliability of your web scraping projects. By utilizing these techniques effectively, you can enhance your scraping performance and gather data more efficiently.
Save time with the Bardeen scraper. Automate routine scraping tasks without coding.
Tools and Frameworks to Streamline Web Scraping
Numerous tools and frameworks are available to simplify the web scraping process, each with unique features and capabilities. Here are some popular options for web scraping:
- Scrapy: An open-source Python framework that provides a complete package for extracting data from websites. It offers built-in support for handling requests, parsing HTML/XML, and storing scraped data.
- Beautiful Soup: A Python library for parsing HTML and XML documents. It simplifies navigating and searching the parsed data, making it easier to extract specific elements.
- Puppeteer: A Node.js library that provides a high-level API to control headless Chrome or Chromium browsers. It enables scraping dynamic websites that heavily rely on JavaScript.
- Selenium: A web testing framework that can also be used for web scraping. It allows you to automate interactions with web pages, making it suitable for scraping complex websites.
In addition to these frameworks, there are cloud-based scraping solutions and tools that provide user-friendly interfaces and require minimal coding:
- Octoparse: A powerful web scraping tool with a visual interface for designing and running scraping tasks. It supports data extraction from various websites and offers features like pagination handling and scheduled scraping.
- ParseHub: Another user-friendly web scraping tool that allows you to extract data without coding. It provides a point-and-click interface for selecting elements and supports handling dynamic content and infinite scrolling.
- Scraper API: A web scraping API that handles proxy rotation, browser rendering, and CAPTCHAs. It allows you to scrape websites using simple API requests without worrying about the underlying infrastructure.
When choosing a web scraping tool or framework, consider factors such as ease of use, scalability, and the specific requirements of your scraping project. It's also essential to be mindful of the legal and ethical aspects of web scraping and ensure compliance with website terms of service.
Automate Your Scraper Tasks with Bardeen
While the article above provides valuable insights on how to web scrape faster using Python techniques, automating the scraping process can significantly streamline your data collection efforts. Bardeen with its Scraper integration can be a game-changer for those looking to automate their web scraping tasks efficiently. Automating web scraping not only saves time but can also perform data extraction around the clock, ensuring you have access to the most up-to-date information without manual intervention.
Here are some examples of how Bardeen can automate your web scraping tasks:
- Extract information from websites in Google Sheets using BardeenAI: This playbook enables automatic extraction of any information from websites directly into a Google Sheet, streamlining the data collection process for further analysis.
- Extract and Summarize Webpage Articles to Text: Perfect for content researchers and creators, this playbook scrapes webpage articles and summarizes them, making it easier to digest and utilize large amounts of information.
- Get keywords and a summary from any website save it to Google Sheets: Automate the extraction of key insights, such as keywords and summaries from websites, and store them in Google Sheets for easy access and analysis.
By leveraging these playbooks, you can significantly enhance your web scraping efficiency and effectiveness. Start automating with Bardeen today by downloading the app at Bardeen.ai/download
Learn how to find or recover an iCloud email using a phone number through Apple ID recovery, device checks, and email searches.
Learn how to find someone's email on TikTok through their bio, social media, Google, and email finder tools. A comprehensive guide for efficient outreach.
Learn how to find a YouTube channel's email for business or collaborations through direct checks, email finder tools, and alternative strategies.
Learn how to find emails on Instagram through direct profile checks or tools like Swordfish AI. Discover methods for efficient contact discovery.
Learn why you can't find Reddit users by email due to privacy policies and discover 3 indirect methods to connect with them.
Learn how to find someone's email address for free using reverse email lookup, email lookup tools, and social media searches. A comprehensive guide.
Your proactive teammate — doing the busywork to save you time
Integrate your apps and websites
Use data and events in one app to automate another. Bardeen supports an increasing library of powerful integrations.
Perform tasks & actions
Bardeen completes tasks in apps and websites you use for work, so you don't have to - filling forms, sending messages, or even crafting detailed reports.
Combine it all to create workflows
Workflows are a series of actions triggered by you or a change in a connected app. They automate repetitive tasks you normally perform manually - saving you time.
Don't just connect your apps, automate them.
200,000+ users and counting use Bardeen to eliminate repetitive tasks